Native Ads
Native Ads allow you to create a better ad experience by blending the ad directly into your app or game, including customizing it to match your UI to a great extent.
Benefits of Native Ads
- Native ads have better eCPMs than Banners, almost comparable to interstitials.
- The use of Native Ads as opposed to other ad types has been shown through multiple experiments to positively impact retention!
You should finish these steps before releasing.
Sizes
A native ad can be displayed in different sizes. The following sizes are available:
Size | Description | Availability |
---|---|---|
Small | The size is customisable with width x height ratio of 3:1 | Phones and Tablets |
Medium | The size is customisable with width x height ratio of 6:5 | Phones and Tablets |
Load and display the ad
You can show the ad using this code, but we recommend using the ad events as you’ll be able to show the ad once it’s loaded. You can check the full script provided below.
- Unity
- Java
- Kotlin
- Swift
- Objective-C
- Godot
- Flutter
Yodo1U3dNativeAdView nativeAdView = new Yodo1U3dNativeAdView(Yodo1U3dNativeAdPosition.NativeTop | Yodo1U3dNativeAdPosition.NativeLeft, 0, 0, 360, 300);
nativeAdView.LoadAd();
To show an ad -
nativeAdView.Show();
To hide an ad -
nativeAdView.Hide();
Positions
Vertical Alignment
The three options for vertical placements of native ads are
- Native Top (
Yodo1U3dNativeAdPosition.NativeTop
) - Native Bottom (
Yodo1U3dNativeAdPosition.NativeBottom
) - Native Vertical Center(
Yodo1U3dNativeAdPosition.NativeVerticalCenter
)
Horizontal Alignment
The three options for horizontal placements of native ads are
- Native Top (
Yodo1U3dNativeAdPosition.NativeLeft
) - Native Bottom (
Yodo1U3dNativeAdPosition.NativeRight
) - Native Vertical Center(
Yodo1U3dNativeAdPosition.NativeHorizontalCenter
)
nativeAdView = new Yodo1U3dNativeAdView(Yodo1U3dNativeAdPosition.NativeTop | Yodo1U3dNativeAdPosition.NativeLeft, 0, 0, 900, 750);
// xml
<com.yodo1.mas.nativeads.Yodo1MasNativeAdView
android:id="@+id/native"
android:layout_width="match_parent"
android:layout_height="300dp"/>
Yodo1MasNativeAdView nativeAdView = findViewById(R.id.native);
nativeAdView.loadAd();
// code
Yodo1MasNativeAdView nativeAdView = new Yodo1MasNativeAdView(this)
layout.addView(nativeAdView, new LayoutParams(LayoutParams.MATCH_PARENT, 300))
nativeAdView.loadAd();
// xml
<com.yodo1.mas.nativeads.Yodo1MasNativeAdView
android:id="@+id/native"
android:layout_width="match_parent"
android:layout_height="300dp"/>
val nativeAdView: Yodo1MasNativeAdView = findViewById(R.id.native);
nativeAdView.loadAd()
// code
val nativeAdView = Yodo1MasNativeAdView(this)
layout.addView(nativeAdView, LayoutParams(LayoutParams.MATCH_PARENT, 300))
nativeAdView.loadAd()
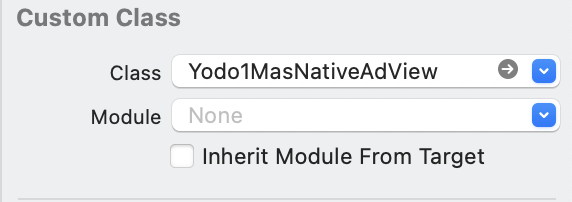
// xib
// Drag a View onto your XIB file and set the Custom Class to Yodo1MasNativeAdView
@IBOutlet weak var nativeAdView: Yodo1MasNativeAdView!
nativeAdView.loadAd()
// code
let nativeAdView = Yodo1MasNativeAdView(frame: CGRect(x: 0, y: 0, width: 320, height: 50))
self.view.addSubview(nativeAdView)
nativeAdView.loadAd()
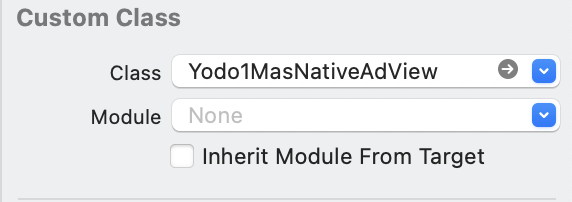
// xib
// Drag a View onto your XIB file and set the Custom Class to Yodo1MasNativeAdView
@property (weak, nonatomic) IBOutlet Yodo1MasNativeAdView *nativeAdView;
[_nativeAdView loadAd];
// code
Yodo1MasNativeAdView *nativeAdView = [[Yodo1MasNativeAdView alloc] initWithFrame:CGRectMake(0, 0, 320, 50)];
[self.view addSubview:nativeAdView];
[nativeAdView loadAd];
// Native ads are displayed automatically
Yodo1MASNativeAd(
size: NativeSize.NativeLarge,
backgroundColor: "BLACK",
),
Configure the ad events
The MAS SDK fires several events to inform you of ad availability.
- Unity
- Java
- Kotlin
- Swift
- Objective-C
- Godot
- Flutter
using Yodo1.MAS;
public class NativeAdLoader : MonoBehaviour
{
Yodo1U3dNativeAdView nativeAdView = null;
private void Start()
{
nativeAdView = new Yodo1U3dNativeAdView(Yodo1U3dNativeAdPosition.NativeTop | Yodo1U3dNativeAdPosition.NativeLeft, 0, 0, 360, 300);
SetupEventCallbacks();
LoadNativeAd();
}
private void SetupEventCallbacks()
{
nativeAdView.OnAdLoadedEvent += OnNativeAdLoadedEvent;
nativeAdView.OnAdFailedToLoadEvent += OnNativeAdLoadFailedEvent;
}
private void LoadNativeAd()
{
nativeAdView.SetAdPlacement("Your placement id");
nativeAdView.LoadAd();
}
private void OnNativeAdLoadedEvent(Yodo1U3dNativeAdView ad)
{
// Code to be executed when an ad finishes loading.
}
private void OnNativeAdLoadFailedEvent(Yodo1U3dNativeAdView ad, Yodo1U3dAdError adError)
{
// Code to be executed when an ad request fails.
}
}
public class NativeActivity extends Activity implements Yodo1MasNativeAdListener {
private Yodo1MasNativeAdView nativeAdView;
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_native);
nativeAdView = this.findViewById(R.id.native);
nativeAdView.setAdListener(this)
nativeAdView.loadAd();
}
@Override
public void onNativeAdLoaded(Yodo1MasNativeAdView nativeAdView) {
// Code to be executed when an ad finishes loading.
}
@Override
public void onNativeAdFailedToLoad(Yodo1MasNativeAdView nativeAdView, Yodo1MasError error) {
// Code to be executed when an ad request fails.
}
}
class NativeActivity: Activity(), Yodo1MasNativeAdListener {
private lateinit var nativeAdView: Yodo1MasNativeAdView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_native)
nativeAdView = this.findViewById(R.id.native)
nativeAdView.setAdListener(this)
nativeAdView.loadAd()
}
override fun onNativeAdLoaded(nativeAdView: Yodo1MasNativeAdView) {
// Code to be executed when an ad finishes loading.
}
override fun onNativeAdFailedToLoad(nativeAdView: Yodo1MasNativeAdView, error: Yodo1MasError) {
// Code to be executed when an ad request fails.
}
}
class NativeController: UIViewController {
@IBOutlet weak var nativeAd: Yodo1MasNativeAdView!
override func viewDidLoad() {
super.viewDidLoad()
// Show ad with placement name, like app_start, level_end
nativeAd.setAdPlacement("Your placement id")
nativeAd.adDelegate = self
nativeAd.loadAd()
}
}
extension NativeController: Yodo1MasNativeAdViewDelegate {
func onNativeAdLoaded(_ adView: Yodo1MasNativeAdView) {
// Code to be executed when an ad finishes loading.
}
func onNativeAdFailed(toLoad adView: Yodo1MasNativeAdView, withError error: Yodo1MasError) {
// Code to be executed when an ad request fails.
}
}
@interface NativeViewController ()<Yodo1MasNativeAdViewDelegate>
@property (weak, nonatomic) IBOutlet Yodo1MasNativeAdView *nativeAdView
@end
@implementation NativeViewController
- (void)viewDidLoad {
[super viewDidLoad];
_nativeAdView.adDelegate = self;
// Show ad with placement name, like app_start, level_end
[_nativeAdView setAdPlacement:@"Your placement id"];
[_nativeAdView loadAd];
}
#pragma mark - Yodo1MasNativeAdViewDelegate
- (void)onNativeAdLoaded:(Yodo1MasNativeAdView *)adView {
// Code to be executed when an ad finishes loading.
}
- (void)onNativeAdFailedToLoad:(Yodo1MasNativeAdView *)adView withError:(Yodo1MasError *)error {
// Code to be executed when an ad request fails.
}
@end
To show App Open Ads, just enable the AppOpenAds checkbox in the editor as shown in the screenshot.
Note : App Open Ads option will only work once the game is live.
Yodo1MASNativeAd(
size: NativeSize.NativeLarge,
backgroundColor: "BLACK",
onLoad: () => print('Native Ad loaded:'),
onClosed: () => print('Native Ad clicked:'),
onLoadFailed: (message) =>
print('Native Ad $message'),
),