Banner Ads
Banner ad units display rectangular ads that occupy a portion of an app's layout. They can refresh automatically after a set period. This means users will view a new ad regularly, even if they stay on the same screen in your app. They're also the simplest ad format to implement.
Benefits of Banner Ads
- Banner Ads are among the oldest advertisements and can make instant impressions at a relatively low cost.
- Banner Ads offer a quick, easy, and cost-effective way to generate immediate interest.
- Banner Ads can pique viewers' curiosity and help retain them by promoting brand awareness.
Supported size
SIZE | DESCRIPTION | AVAILABILITY | ADSIZE CONSTANT |
---|---|---|---|
320×50 | Standard Banner | Phones and Tablets | Banner |
320×100 | Large Banner | Phones and Tablets | LargeBanner |
300×250 | IAB Medium Rectangle | Phones and Tablets | IABMediumRectangle |
Full-screen width x Adaptive height | Adaptive banner | Phones and Tablets | AdaptiveBanner |
Screen width x 32/50/90 | Smart banner | Phones and Tablets | SmartBanner |
You should finish these steps before releasing.
Load and Display the ad
You can show the ad using this code, but we recommend using the ad events as you’ll be able to show the ad once it’s loaded. You can check the full script provided below.
- Unity
- Java
- Kotlin
- Swift
- Objective-C
- Godot
- Cocos2d
- React Native
Yodo1U3dBannerAdView bannerAdView = new Yodo1U3dBannerAdView(Yodo1U3dBannerAdSize.Banner, Yodo1U3dBannerAdPosition.BannerBottom | Yodo1U3dBannerAdPosition.BannerHorizontalCenter);
bannerAdView.LoadAd();
bannerAdView.Show();
// xml
<com.yodo1.mas.banner.Yodo1MasBannerAdView
android:id="@+id/banner"
android:layout_width="320dp"
android:layout_height="50dp"
masads:adSize="Banner" />
Yodo1MasBannerAdView bannerAdView = findViewById(R.id.banner);
bannerAdView.loadAd();
// code
Yodo1MasBannerAdView bannerAdView = new Yodo1MasBannerAdView(this);
bannerAdView.setAdSize(Yodo1MasBannerAdSize.Banner);
layout.addView(bannerAdView, new LayoutParams(320, 50));
bannerAdView.loadAd();
// xml
<com.yodo1.mas.banner.Yodo1MasBannerAdView
android:id="@+id/banner"
android:layout_width="320dp"
android:layout_height="50dp"
masads:adSize="Banner" />
val bannerAdView: Yodo1MasBannerAdView = findViewById(R.id.banner)
bannerAdView.loadAd()
// code
val bannerAdView = Yodo1MasBannerAdView(this)
bannerAdView.setAdSize(Yodo1MasBannerAdSize.Banner)
layout.addView(bannerAdView, LayoutParams(320, 50))
bannerAdView.loadAd()
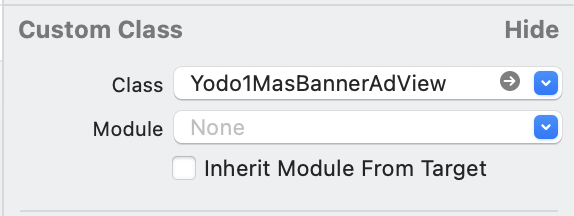
// xib
// Drag a View onto your XIB file and set the Custom Class to Yodo1MasBannerAdView
@IBOutlet weak var bannerAdView: Yodo1MasBannerAdView!
bannerAdView.loadAd()
// code
let bannerAdView = Yodo1MasBannerAdView(frame: CGRect(x: 0, y: 0, width: 320, height: 50))
self.view.addSubview(bannerAdView)
bannerAdView.loadAd()
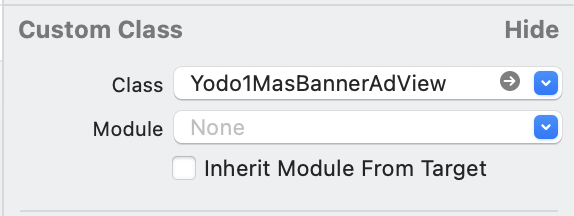
// xib
// Drag a View onto your XIB file and set the Custom Class to Yodo1MasBannerAdView
@property (weak, nonatomic) IBOutlet Yodo1MasBannerAdView *bannerAdView;
[_bannerAdView loadAd];
// code
Yodo1MasBannerAdView *bannerAdView = [[Yodo1MasBannerAdView alloc] initWithFrame:CGRectMake(0, 0, 320, 50)];
[self.view addSubview:bannerAdView];
[bannerAdView loadAd];
yodo1mas.load_banner_ad("Banner","RIGHT","TOP")
Yodo1Ads.getInstance().loadBannerAds("Banner","RIGHT","TOP");
Yodo1Ads.getInstance().showBannerAds();
import { findNodeHandle } from 'react-native';
const reactTag = findNodeHandle(ref);
Yodo1MASAds.showBannerAds(reactTag);
Configure the ad events
The MAS SDK fires several events to inform you of ad availability.
- Unity
- Java
- Kotlin
- Swift
- Objective-C
- Godot
- React Native
using Yodo1.MAS;
public class BannerAdLoader : MonoBehaviour
{
Yodo1U3dBannerAdView bannerAdView = null;
private void Start()
{
bannerAdView = new Yodo1U3dBannerAdView(Yodo1U3dBannerAdSize.Banner, Yodo1U3dBannerAdPosition.BannerBottom | Yodo1U3dBannerAdPosition.BannerHorizontalCenter);
SetupEventCallbacks();
LoadBannerAd();
}
private void SetupEventCallbacks()
{
bannerAdView.OnAdLoadedEvent += OnBannerAdLoadedEvent;
bannerAdView.OnAdFailedToLoadEvent += OnBannerAdLoadFailedEvent;
bannerAdView.OnAdOpenedEvent += OnBannerAdOpenedEvent;
bannerAdView.OnAdFailedToOpenEvent += OnBannerAdOpenFailedEvent;
bannerAdView.OnAdClosedEvent += OnBannerAdClosedEvent;
}
private void LoadBannerAd()
{
bannerAdView.SetAdPlacement("Your placement ID");
bannerAdView.LoadAd();
}
private void OnBannerAdLoadedEvent(Yodo1U3dBannerAdView ad)
{
// Code to be executed when an ad finishes loading.
}
private void OnBannerAdLoadFailedEvent(Yodo1U3dBannerAdView ad, Yodo1U3dAdError adError)
{
// Code to be executed when an ad request fails.
}
private void OnBannerAdOpenedEvent(Yodo1U3dBannerAdView ad)
{
// Code to be executed when an ad opened
}
private void OnBannerAdOpenFailedEvent(Yodo1U3dBannerAdView ad, Yodo1U3dAdError adError)
{
// Code to be executed when an ad open fails.
}
private void OnBannerAdClosedEvent(Yodo1U3dBannerAdView ad)
{
// Code to be executed when the ad closed
}
}
public class BannerActivity extends Activity implements Yodo1MasBannerAdListener {
private Yodo1MasBannerAdView bannerAdView;
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_banner);
bannerAdView = this.findViewById(R.id.banner);
bannerAdView.setAdListener(this);
bannerAdView.loadAd();
}
public void onBannerAdLoaded(Yodo1MasBannerAdView bannerAdView) {
// Code to be executed when an ad finishes loading.
}
@Override
public void onBannerAdFailedToLoad(Yodo1MasBannerAdView bannerAdView, @NonNull Yodo1MasError error) {
// Code to be executed when an ad request fails.
}
@Override
public void onBannerAdOpened(Yodo1MasBannerAdView bannerAdView) {
// Code to be executed when an ad opened
}
@Override
public void onBannerAdFailedToOpen(Yodo1MasBannerAdView bannerAdView, @NonNull Yodo1MasError error) {
// Code to be executed when an ad open fails.
}
@Override
public void onBannerAdClosed(Yodo1MasBannerAdView bannerAdView) {
// Code to be executed when the ad closed
}
}
class BannerActivity: Activity(), Yodo1MasBannerAdListener {
private lateinit var bannerAdView: Yodo1MasBannerAdView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_banner)
bannerAdView = this.findViewById(R.id.banner)
bannerAdView.setAdListener(this)
bannerAdView.loadAd()
}
override fun onBannerAdLoaded(bannerAdView: Yodo1MasBannerAdView) {
// Code to be executed when an ad finishes loading.
}
override fun onBannerAdFailedToLoad(bannerAdView: Yodo1MasBannerAdView, error: Yodo1MasError) {
// Code to be executed when an ad request fails.
}
override fun onBannerAdOpened(bannerAdView: Yodo1MasBannerAdView) {
// Code to be executed when an ad opened
}
override fun onBannerAdFailedToOpen(bannerAdView: Yodo1MasBannerAdView, error: Yodo1MasError) {
// Code to be executed when an ad open fails.
}
override fun onBannerAdClosed(bannerAdView: Yodo1MasBannerAdView) {
// Code executed when getting rewards
}
}
class BannerController: UIViewController {
@IBOutlet weak var bannerAd: Yodo1MasBannerAdView!
override func viewDidLoad() {
super.viewDidLoad()
// Show ad with placement name, like app_start, level_end
bannerAd.setAdPlacement("Your placement ID")
bannerAd.adDelegate = self
bannerAd.loadAd()
}
}
extension BannerController: Yodo1MasBannerAdViewDelegate {
func onBannerAdLoaded(_ adView: Yodo1MasBannerAdView) {
// Code to be executed when an ad finishes loading.
}
func onBannerAdFailed(toLoad adView: Yodo1MasBannerAdView, withError error: Yodo1MasError) {
// Code to be executed when an ad request fails.
}
func onBannerAdOpened(_ adView: Yodo1MasBannerAdView) {
// Code to be executed when an ad opened
}
func onBannerAdFailed(toOpen adView: Yodo1MasBannerAdView, withError error: Yodo1MasError) {
// Code to be executed when an ad open fails.
}
func onBannerAdClosed(_ adView: Yodo1MasBannerAdView) {
// Code executed when getting rewards
}
}
@interface BannerViewController ()<Yodo1MasBannerAdViewDelegate>
@property (weak, nonatomic) IBOutlet Yodo1MasBannerAdView *bannerAdView
@end
@implementation BannerViewController
- (void)viewDidLoad {
[super viewDidLoad];
_bannerAdView.adDelegate = self;
// Show ad with placement name, like app_start, level_end
[_bannerAdView setAdPlacement:@"Your placement ID"];
[_bannerAdView setAdSize:Yodo1MasBannerAdSizeBanner];
[_bannerAdView loadAd];
}
#pragma mark - Yodo1MasBannerAdViewDelegate
- (void)onBannerAdLoaded:(Yodo1MasBannerAdView *)adView {
// Code to be executed when an ad finishes loading.
}
- (void)onBannerAdFailedToLoad:(Yodo1MasBannerAdView *)adView withError:(Yodo1MasError *)error {
// Code to be executed when an ad request fails.
}
- (void)onBannerAdOpened:(Yodo1MasBannerAdView *)adView {
// Code to be executed when an ad opened
}
- (void)onBannerAdFailedToOpen:(Yodo1MasBannerAdView *)adView withError:(Yodo1MasError *)error {
// Code to be executed when an ad open fails.
}
- (void)onBannerAdClosed:(Yodo1MasBannerAdView *)adView {
// Code to be executed when the ad closed
}
@end
func _on_Yodo1Mas_banner_ad_loaded():
func _on_Yodo1Mas_banner_failed_ad_loaded():
func _on_Yodo1Mas_banner_ad_opened():
func _on_Yodo1Mas_banner_ad_failed_opened():
func _on_Yodo1Mas_banner_ad_closed():
const handleEvents = ({value}) => {
__DEV__ && console.log(`Event: ${value}`);
switch (value) {
case 'onBannerAdLoaded':
// Handle the event when the ad is loaded
break;
case 'onBannerAdOpened':
// Handle the event when the ad is opened
break;
case 'onBannerAdClosed':
// Handle the event when the ad is closed
break;
case 'onBannerAdFailedToLoad':
// Ad failed to load, let's try again after a delay
break;
case 'onBannerAdFailedToOpen':
// Ad failed to open, let's try again after a delay
break;
}
};
export const registerYodo1MAS = () => {
const eventEmitter = new NativeEventEmitter(Yodo1MASAds);
const eventListener = eventEmitter.addListener('adEvent', handleEvents);
// If needed, pass CCPA, COPPA, GDPR values in the initSDK method
Yodo1MASAds.initMasSdk(true, true, true);
return eventListener;
};
Impression-Level User Revenue
Starting in SDK version 4.13.2, you can access impression-level user revenue data on the client side. You can use this data to compare different sources and campaigns.
You can share impression-level ad revenue data with your mobile measurement partner of choice.
You can retrieve the revenue amount in all ad lifecycle callbacks. The following example shows how to do this within callback:
- Unity
- Java
- Kotlin
- Swift
- Objective-C
bannerAdView.OnAdPayRevenueEvent += OnBannerAdPayRevenueEvent;
private void OnBannerAdPayRevenueEvent(Yodo1U3dBannerAdView ad, Yodo1U3dAdValue adValue)
{
double revenue = adValue.Revenue;
string currency = adValue.Currency;
}
bannerAdView.setAdRevenueListener(new Yodo1MasBannerAdRevenueListener() {
@Override
public void onBannerAdPayRevenue(Yodo1MasBannerAdView view, Yodo1MasAdValue adValue) {
double revenue = adValue.getRevenue();
String currency = adValue.getCurrency();
}
});
bannerAdView.setAdRevenueListener(Yodo1MasBannerAdRevenueListener { view, adValue ->
val revenue = adValue.revenue
val currency = adValue.currency
})
_bannerAdView.adRevenueDelegate = self
func onBannerAdPayRevenue(_ ad: Yodo1MasBannerAdView, _ adValue: Yodo1MasAdValue)
{
let revenue = adValue.revenue
let currency = adValue.currency
}
_bannerAdView.adRevenueDelegate = self;
#pragma mark - Yodo1MasBannerAdRevenueDelegate
- (void)onBannerAdPayRevenue:(Yodo1MasBannerAdView *)ad withAdValue:(Yodo1MasAdValue *)adValue {
double revenue = adValue.revenue;
NSString *currency = adValue.currency;
}
You can also retrieve a precision evaluation for the revenue value, as shown in the following example:
- Unity
- Java
- Kotlin
- Swift
- Objective-C
string revenuePrecision = adValue.RevenuePrecision;
String revenuePrecision = adValue.getRevenuePrecision();
val revenuePrecision = adValue.revenuePrecision
let revenuePrecision = adValue.revenuePrecision
NSString *revenuePrecision = adValue.revenuePrecision;