Native Ads
Native Ads allow you to create a better ad experience by blending the ad directly into your app or game, including customizing it to match your UI to a great extent.
Benefits of Native Ads
- Native ads have better eCPMs than Banners, almost comparable to interstitials.
- The use of Native Ads as opposed to other ad types has been shown through multiple experiments to positively impact retention!
You should finish these steps before releasing.
Sizes
A native ad can be displayed in different sizes. The following sizes are available:
Size | Description | Availability |
---|---|---|
Small | The size is customisable with width x height ratio of 3:1 | Phones and Tablets |
Medium | The size is customisable with width x height ratio of 6:5 | Phones and Tablets |
Load and display the ad
You can show the ad using this code, but we recommend using the ad events as you’ll be able to show the ad once it’s loaded. You can check the full script provided below.
- Unity
- Java
- Kotlin
- Swift
- Objective-C
- Godot
- React Native
Yodo1U3dNativeAdView nativeAdView = new Yodo1U3dNativeAdView(Yodo1U3dNativeAdPosition.NativeTop | Yodo1U3dNativeAdPosition.NativeLeft, 0, 0, 360, 300);
nativeAdView.LoadAd();
To show an ad -
nativeAdView.Show();
To hide an ad -
nativeAdView.Hide();
Positions
Vertical Alignment
The three options for vertical placements of native ads are
- Native Top (
Yodo1U3dNativeAdPosition.NativeTop
) - Native Bottom (
Yodo1U3dNativeAdPosition.NativeBottom
) - Native Vertical Center(
Yodo1U3dNativeAdPosition.NativeVerticalCenter
)
Horizontal Alignment
The three options for horizontal placements of native ads are
- Native Top (
Yodo1U3dNativeAdPosition.NativeLeft
) - Native Bottom (
Yodo1U3dNativeAdPosition.NativeRight
) - Native Vertical Center(
Yodo1U3dNativeAdPosition.NativeHorizontalCenter
)
nativeAdView = new Yodo1U3dNativeAdView(Yodo1U3dNativeAdPosition.NativeTop | Yodo1U3dNativeAdPosition.NativeLeft, 0, 0, 900, 750);
// xml
<com.yodo1.mas.nativeads.Yodo1MasNativeAdView
android:id="@+id/native"
android:layout_width="match_parent"
android:layout_height="300dp"/>
Yodo1MasNativeAdView nativeAdView = findViewById(R.id.native);
nativeAdView.loadAd();
// code
Yodo1MasNativeAdView nativeAdView = new Yodo1MasNativeAdView(this)
layout.addView(nativeAdView, new LayoutParams(LayoutParams.MATCH_PARENT, 300))
nativeAdView.loadAd();
// xml
<com.yodo1.mas.nativeads.Yodo1MasNativeAdView
android:id="@+id/native"
android:layout_width="match_parent"
android:layout_height="300dp"/>
val nativeAdView: Yodo1MasNativeAdView = findViewById(R.id.native);
nativeAdView.loadAd()
// code
val nativeAdView = Yodo1MasNativeAdView(this)
layout.addView(nativeAdView, LayoutParams(LayoutParams.MATCH_PARENT, 300))
nativeAdView.loadAd()
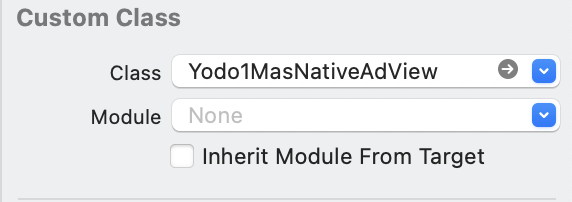
// xib
// Drag a View onto your XIB file and set the Custom Class to Yodo1MasNativeAdView
@IBOutlet weak var nativeAdView: Yodo1MasNativeAdView!
nativeAdView.loadAd()
// code
let nativeAdView = Yodo1MasNativeAdView(frame: CGRect(x: 0, y: 0, width: 320, height: 50))
self.view.addSubview(nativeAdView)
nativeAdView.loadAd()
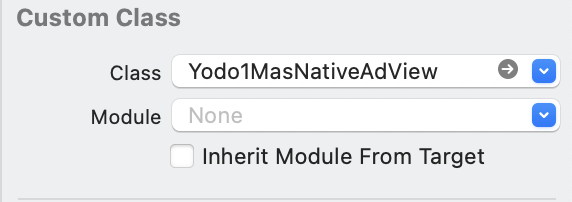
// xib
// Drag a View onto your XIB file and set the Custom Class to Yodo1MasNativeAdView
@property (weak, nonatomic) IBOutlet Yodo1MasNativeAdView *nativeAdView;
[_nativeAdView loadAd];
// code
Yodo1MasNativeAdView *nativeAdView = [[Yodo1MasNativeAdView alloc] initWithFrame:CGRectMake(0, 0, 320, 50)];
[self.view addSubview:nativeAdView];
[nativeAdView loadAd];
// Native ads are displayed automatically
import { findNodeHandle } from 'react-native';
const reactTag = findNodeHandle(ref);
Yodo1MASAds.showNativeAds(reactTag);
Configure the ad events
The MAS SDK fires several events to inform you of ad availability.
- Unity
- Java
- Kotlin
- Swift
- Objective-C
- Godot
- React Native
using Yodo1.MAS;
public class NativeAdLoader : MonoBehaviour
{
Yodo1U3dNativeAdView nativeAdView = null;
private void Start()
{
nativeAdView = new Yodo1U3dNativeAdView(Yodo1U3dNativeAdPosition.NativeTop | Yodo1U3dNativeAdPosition.NativeLeft, 0, 0, 360, 300);
SetupEventCallbacks();
LoadNativeAd();
}
private void SetupEventCallbacks()
{
nativeAdView.OnAdLoadedEvent += OnNativeAdLoadedEvent;
nativeAdView.OnAdFailedToLoadEvent += OnNativeAdLoadFailedEvent;
}
private void LoadNativeAd()
{
nativeAdView.SetAdPlacement("Your placement ID");
nativeAdView.LoadAd();
}
private void OnNativeAdLoadedEvent(Yodo1U3dNativeAdView ad)
{
// Code to be executed when an ad finishes loading.
}
private void OnNativeAdLoadFailedEvent(Yodo1U3dNativeAdView ad, Yodo1U3dAdError adError)
{
// Code to be executed when an ad request fails.
}
}
public class NativeActivity extends Activity implements Yodo1MasNativeAdListener {
private Yodo1MasNativeAdView nativeAdView;
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_native);
nativeAdView = this.findViewById(R.id.native);
nativeAdView.setAdListener(this)
nativeAdView.loadAd();
}
@Override
public void onNativeAdLoaded(Yodo1MasNativeAdView nativeAdView) {
// Code to be executed when an ad finishes loading.
}
@Override
public void onNativeAdFailedToLoad(Yodo1MasNativeAdView nativeAdView, Yodo1MasError error) {
// Code to be executed when an ad request fails.
}
}
class NativeActivity: Activity(), Yodo1MasNativeAdListener {
private lateinit var nativeAdView: Yodo1MasNativeAdView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_native)
nativeAdView = this.findViewById(R.id.native)
nativeAdView.setAdListener(this)
nativeAdView.loadAd()
}
override fun onNativeAdLoaded(nativeAdView: Yodo1MasNativeAdView) {
// Code to be executed when an ad finishes loading.
}
override fun onNativeAdFailedToLoad(nativeAdView: Yodo1MasNativeAdView, error: Yodo1MasError) {
// Code to be executed when an ad request fails.
}
}
class NativeController: UIViewController {
@IBOutlet weak var nativeAd: Yodo1MasNativeAdView!
override func viewDidLoad() {
super.viewDidLoad()
// Show ad with placement name, like app_start, level_end
nativeAd.setAdPlacement("Your placement ID")
nativeAd.adDelegate = self
nativeAd.loadAd()
}
}
extension NativeController: Yodo1MasNativeAdViewDelegate {
func onNativeAdLoaded(_ adView: Yodo1MasNativeAdView) {
// Code to be executed when an ad finishes loading.
}
func onNativeAdFailed(toLoad adView: Yodo1MasNativeAdView, withError error: Yodo1MasError) {
// Code to be executed when an ad request fails.
}
}
@interface NativeViewController ()<Yodo1MasNativeAdViewDelegate>
@property (weak, nonatomic) IBOutlet Yodo1MasNativeAdView *nativeAdView
@end
@implementation NativeViewController
- (void)viewDidLoad {
[super viewDidLoad];
_nativeAdView.adDelegate = self;
// Show ad with placement name, like app_start, level_end
[_nativeAdView setAdPlacement:@"Your placement ID"];
[_nativeAdView loadAd];
}
#pragma mark - Yodo1MasNativeAdViewDelegate
- (void)onNativeAdLoaded:(Yodo1MasNativeAdView *)adView {
// Code to be executed when an ad finishes loading.
}
- (void)onNativeAdFailedToLoad:(Yodo1MasNativeAdView *)adView withError:(Yodo1MasError *)error {
// Code to be executed when an ad request fails.
}
@end
To show App Open Ads, just enable the AppOpenAds checkbox in the editor as shown in the screenshot.
Note : App Open Ads option will only work once the game is live.
const handleEvents = ({value}) => {
__DEV__ && console.log(`Event: ${value}`);
switch (value) {
case 'onNativeAdLoaded':
// Handle the event when the ad is loaded
break;
case 'onNativeAdFailedToLoad':
// Ad failed to load, let's try again after a delay
break;
}
};
export const registerYodo1MAS = () => {
const eventEmitter = new NativeEventEmitter(Yodo1MASAds);
const eventListener = eventEmitter.addListener('adEvent', handleEvents);
// If needed, pass CCPA, COPPA, GDPR values in the initSDK method
Yodo1MASAds.initMasSdk(true, true, true);
return eventListener;
};
Impression-Level User Revenue
Starting in SDK version 4.13.2, you can access impression-level user revenue data on the client side. You can use this data to compare different sources and campaigns.
You can share impression-level ad revenue data with your mobile measurement partner of choice.
You can retrieve the revenue amount in all ad lifecycle callbacks. The following example shows how to do this within callback:
- Unity
- Java
- Kotlin
- Swift
- Objective-C
nativeAdView.OnAdPayRevenueEvent += OnNativeAdPayRevenueEvent;
private void OnNativeAdPayRevenueEvent(Yodo1U3dBannerAdView ad, Yodo1U3dAdValue adValue)
{
double revenue = adValue.Revenue;
string currency = adValue.Currency;
}
nativeAdView.setAdRevenueListener(new Yodo1MasNativeAdRevenueListener() {
@Override
public void onNativedAdPayRevenue(Yodo1MasNativeAdView view, Yodo1MasAdValue adValue) {
double revenue = adValue.getRevenue();
String currency = adValue.getCurrency();
}
});
nativeAdView.setAdRevenueListener(Yodo1MasNativeAdRevenueListener { view, adValue ->
val revenue = adValue.revenue
val currency = adValue.currency
})
_nativeAdView.adRevenueDelegate = self;
func onNativeAdPayRevenue(_ ad: Yodo1MasNativeAdView, _ adValue: Yodo1MasAdValue)
{
let revenue = adValue.revenue
let currency = adValue.currency
}
_nativeAdView.adRevenueDelegate = self;
#pragma mark - Yodo1MasNativeAdRevenueDelegate
- (void)onNativeAdPayRevenue:(Yodo1MasNativeAdView *)ad withAdValue:(nonnull Yodo1MasAdValue *)adValue {
double revenue = adValue.revenue;
NSString *currency = adValue.currency;
}
You can also retrieve a precision evaluation for the revenue value, as shown in the following example:
- Unity
- Java
- Kotlin
- Swift
- Objective-C
string revenuePrecision = adValue.RevenuePrecision;
String revenuePrecision = adValue.getRevenuePrecision();
val revenuePrecision = adValue.revenuePrecision
let revenuePrecision = adValue.revenuePrecision
NSString *revenuePrecision = adValue.revenuePrecision;