Unity Integration
This page guides you through the process of downloading, importing, and configuring the Yodo1 MAS SDK for Unity.
Prerequisites
To integrate the Yodo1 MAS SDK on Unity, you need to meet the following requirements:
- Unity 2021.3.41f1 or above
- For Android
- Minimum API Level 24 or above
- Target API Level 34 or above
- If you use Proguard, please refer to the documentation.
- For iOS
- iOS 13.0 or above.
- Xcode 16.0 or above
- Cocoapods 1.10.0 or above
Download and Import
Download the SDK
Download the latest SDK package:
Import the Unity Package
- Open your Unity project and import the Unity package
- The files will automatically populate in your project
- For iOS builds, update your iOS resolver settings under External Dependency Manager -> iOS Resolver:
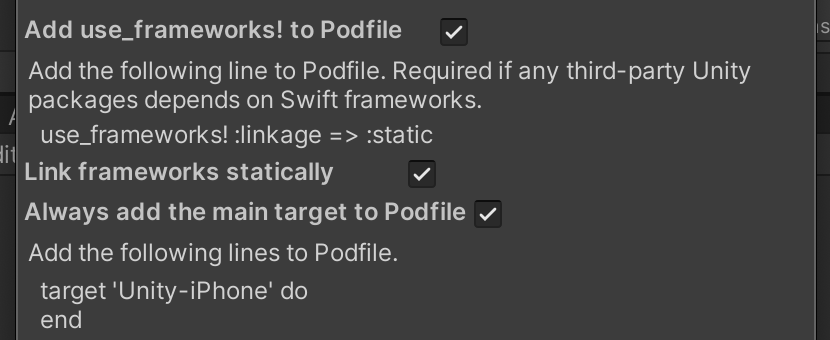
Ad Network Manager
Starting from MAS 4.8.10, we have introduced the Ad Network Manager, allowing dynamic addition or removal of ad networks.
Please contact support for advice on which ad networks are safe to remove without impacting performance.
Configure the SDK
You need to add your Appkey manually in your project if you haven’t added your game’s store link in the MAS dashboard yet. You can find your app key in MAS dashboard.
SDK Settings
- Go to
Yodo1
->MAS Settings
in Unity Editor - Configure your app-key and AdMob key for both Android and iOS platforms
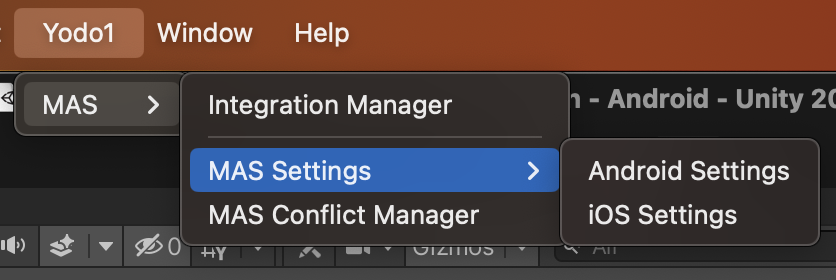
Android settings:
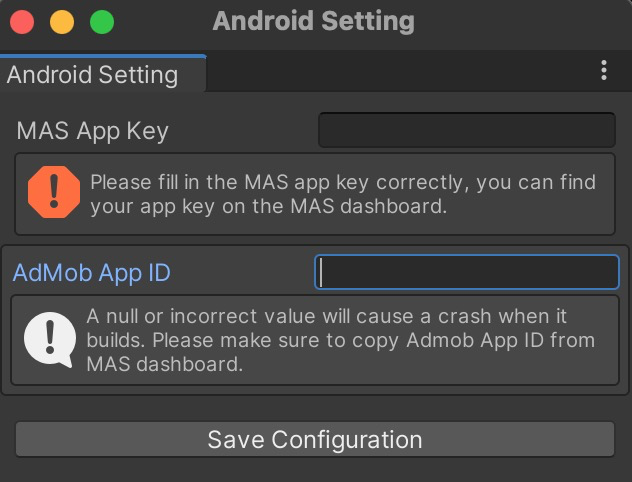
iOS settings:
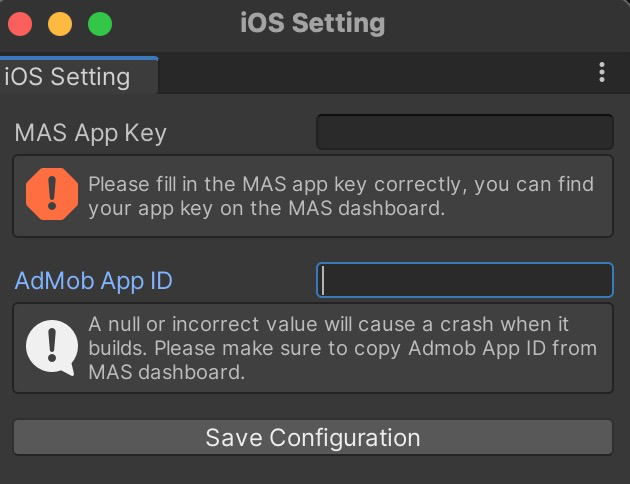
Initialize the SDK
Initialize the SDK in the Awake
method of your MonoBehaviour
class:
using Yodo1.MAS;
public class YourClass : MonoBehaviour
{
void Awake()
{
Yodo1U3dMas.InitializeMasSdk();
}
}
You can check the initialization status using callbacks:
Yodo1U3dMasCallback.OnSdkInitializedEvent += (success, error) =>
{
if (success)
{
Debug.Log("[Yodo1 Mas] The initialization has succeeded");
}
else
{
Debug.Log("[Yodo1 Mas] The initialization has failed with error " + error.ToString());
}
};
Legal Framework Configuration
Configure COPPA
- It's mandatory to use a privacy dialog to comply with store policies and avoid revenue loss
- If you use MAS built-in privacy dialog, COPPA will be configured automatically
- If you prefer using your own privacy dialog, you should check the user's age and set COPPA manually
- You need to set COPPA before initializing MAS
The Children's Online Privacy Protection Act (COPPA) is an American legal framework that safeguards children's privacy. If your app is designed for children under 13 years of age, it must include COPPA compliance.
// For users under 13
Yodo1U3dMas.SetCOPPA(true);
// For users 13 and above
Yodo1U3dMas.SetCOPPA(false);
Configure GDPR
- It's mandatory to use a privacy dialog to comply with store policies and avoid revenue loss
- If you use MAS built-in privacy dialog, GDPR will be configured automatically
- If you prefer using your own privacy dialog, you should check users' age and add an agreement link to get their consent
- You need to set GDPR before initializing MAS
The General Data Protection Regulation (GDPR) applies to users in the European Union (EU) and European Economic Area (EEA). Users under 16 must opt out of data collection.
// For users who consent to data collection
Yodo1U3dMas.SetGDPR(true);
// For users who don't consent or are under 16
Yodo1U3dMas.SetGDPR(false);
Configure CCPA
- It's mandatory to use a privacy dialog to comply with store policies and avoid revenue loss
- If you use MAS built-in privacy dialog, CCPA will be configured automatically
- If you prefer using your own privacy dialog, you should add an agreement link to get users' consent
- You need to set CCPA before initializing MAS
The California Consumer Privacy Act (CCPA) is a legal framework that protects the privacy of California residents in the USA.
// For users who opt out of data collection
Yodo1U3dMas.SetCCPA(true);
// For users who have not opted out
Yodo1U3dMas.SetCCPA(false);