Cocos Integration
This page guides you through the process of downloading, importing, and configuring the Yodo1 MAS SDK for Cocos Creator.
Prerequisites
To integrate the Yodo1 MAS SDK on Cocos Creator, you need to meet the following requirements:
- Cocos Creator 3.0 or above
- For Android:
- Minimum API Level 24 or above
- Target API Level 34 or above
- If you use Proguard, please refer to the documentation
- For iOS:
- iOS 13.0 or above
- Xcode 16.0 or above
- CocoaPods 1.10.0 or above
Download and Setup
- Download Yodo1Ads.ts and add it to your project
- Create a new Node and drop
Yodo1Ads.ts
script on it
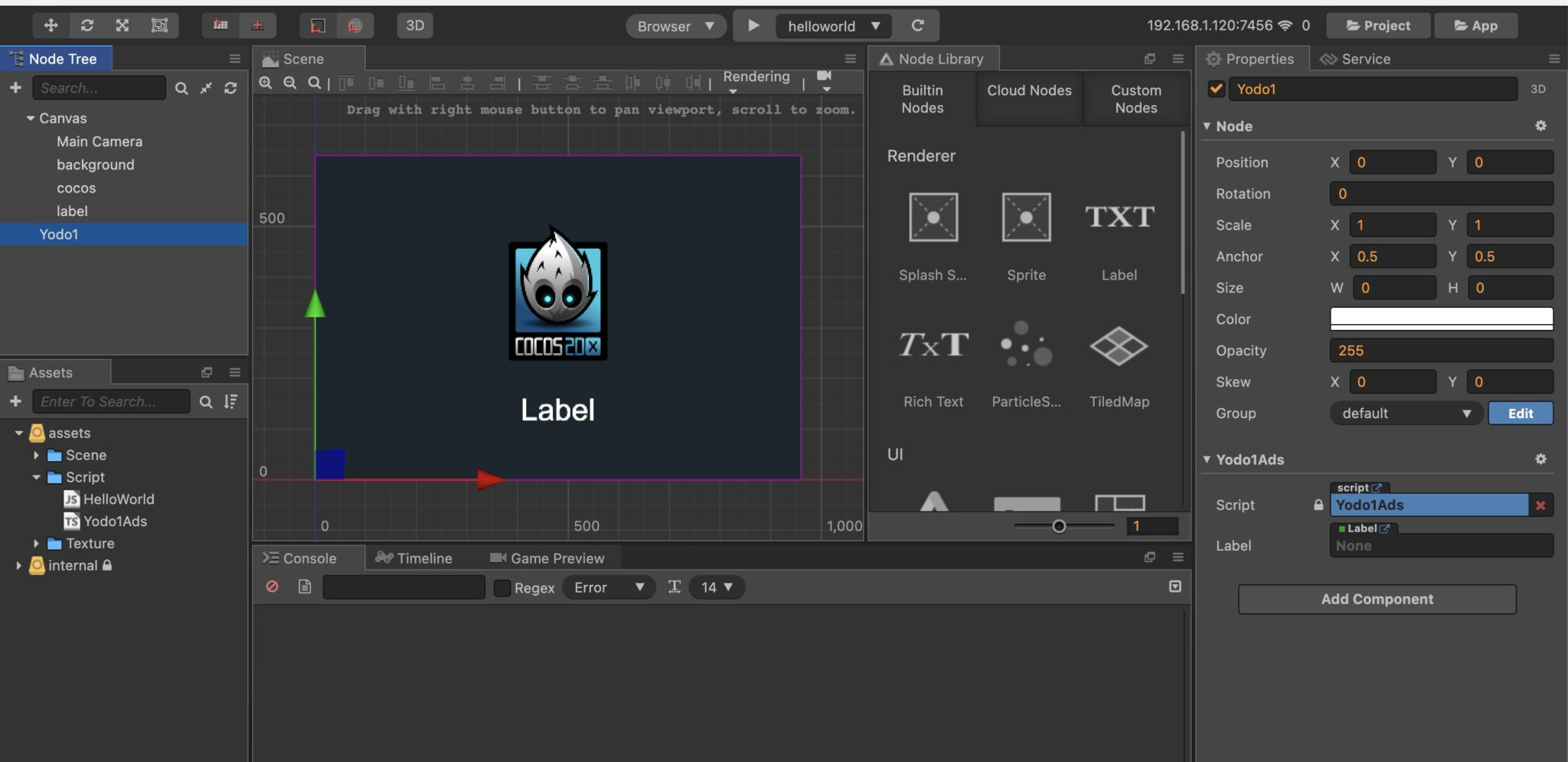
Android Setup
Configure Build Settings
- Generate Android project:
- Go to Project > Build
- Click the Build button
- Open the generated folder in Android Studio:
your_project_folder/build/jsb-default/frameworks/runtime-src/proj.android-studio

Configure Dependencies
- Add repositories to project-level
build.gradle
:
allprojects {
repositories {
google()
jcenter()
mavenCentral()
maven { url "https://artifact.bytedance.com/repository/pangle" }
maven { url "https://android-sdk.is.com" }
maven { url "https://dl-maven-android.mintegral.com/repository/mbridge_android_sdk_oversea" }
maven { url "https://artifactory.bidmachine.io/bidmachine" }
maven { url "https://ysonetwork.s3.eu-west-3.amazonaws.com/sdk/android" }
maven { url "https://repo.pubmatic.com/artifactory/public-repos" }
maven { url "https://bitbucket.org/sdkcenter/sdkcenter/raw/release" }
}
}
- Add dependencies to app-level
build.gradle
:
implementation 'com.yodo1.mas:full:4.15.0'
- Enable MultiDex in app-level
build.gradle
:
defaultConfig {
multiDexEnabled true
}
- Configure
gradle.properties
:
android.useAndroidX=true
android.enableJetifier=true
android.enableDexingArtifactTransform=false
org.gradle.daemon=true
org.gradle.jvmargs=-Xmx2560m
Configure Native Code
- Add AdMob ID to
AndroidManifest.xml
:
<meta-data
android:name="com.google.android.gms.ads.APPLICATION_ID"
android:value="Your_Admob_ID"
/>
- Add Yodo1Ads.java file alongside
AppActivity.java
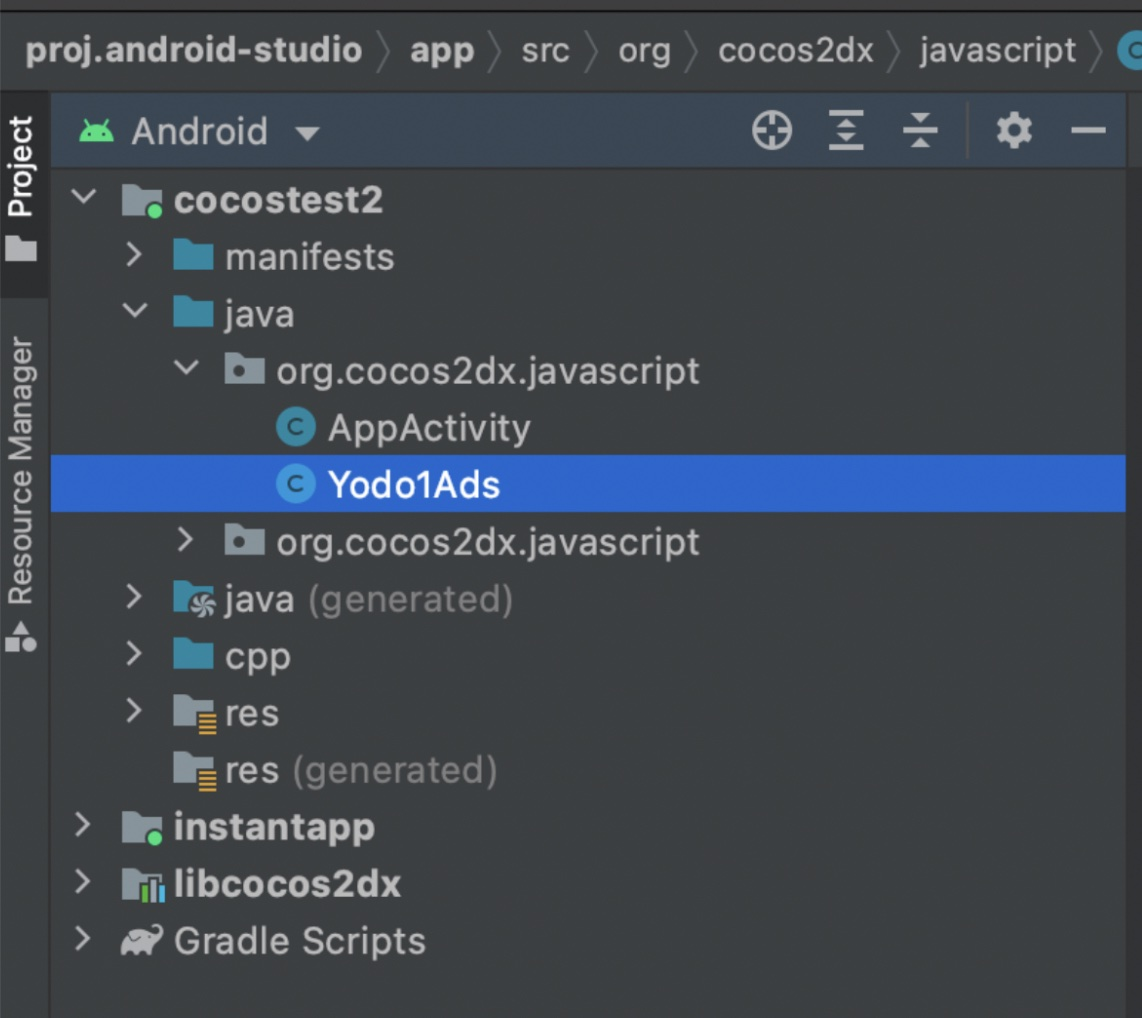
- Update
AppActivity.java
:
public static void initializeSdk(String appKey,boolean isEnabled)
{
Yodo1Ads.initializeSdk(activity, appKey,isEnabled);
}
public static void loadBannerAds(String size,String horizontal,String Vertical)
{
Yodo1Ads.loadBannerAds(size,horizontal,Vertical);
}
public static void hideBannerAds()
{
Yodo1Ads.hideBannerAds();
}
public static void showBannerAds()
{
Yodo1Ads.showBannerAds();
}
public static void initializeInterstitialAds()
{
Yodo1Ads.initializeInterstitialAds();
}
public static void showInterstitialAds()
{
Yodo1Ads.showInterstitialAds();
}
public static void initializeRewardAds()
{
Yodo1Ads.initializeRewardAds();
}
public static void showRewardAds()
{
Yodo1Ads.showRewardAds();
}
public static void setCOPPA(boolean isEnabled)
{
Yodo1Ads.setCOPPA(isEnabled);
}
public static void setGDPR(boolean isEnabled)
{
Yodo1Ads.setGDPR(isEnabled);
}
public static void setCCPA(boolean isEnabled) {
Yodo1Ads.setCCPA(isEnabled);
}
- Create and configure
AppActivity
static object:
iOS Setup
Configure CocoaPods
- Add to your Podfile:
platform :ios, '13.0'
source 'https://cdn.cocoapods.org/'
source 'https://github.com/Yodo1Games/MAS-Spec.git'
target 'cocos2d' do
use_frameworks!
end
target 'cocosiOS-mobile' do
use_frameworks! :linkage => :static
pod 'Yodo1MasFull', '4.11.0'
end
- Install pods:
pod install
Configure Info.plist
- Setup/Remove AppLovin Key
For MAS SDK 4.15.0 or above, remove the AppLovinSdkKey from your app's Info.plist.
For MAS SDK 4.14.2 or below, ensure that the AppLovinSdkKey is added to the Info.plist.
<key>AppLovinSdkKey</key>
<string>xcGD2fy-GdmiZQapx_kUSy5SMKyLoXBk8RyB5u9MVv34KetGdbl4XrXvAUFy0Qg9scKyVTI0NM4i_yzdXih4XE</string>
- Configure App Transport Security:
<key>NSAppTransportSecurity</key>
<dict>
<key>NSAllowsArbitraryLoads</key>
<true/>
</dict>
- Add App Tracking Transparency:
<key>NSUserTrackingUsageDescription</key>
<string>This identifier will be used to deliver personalized ads to you.</string>
Initialize SDK
Add the Yodo1Ads
classes and headers to your project:
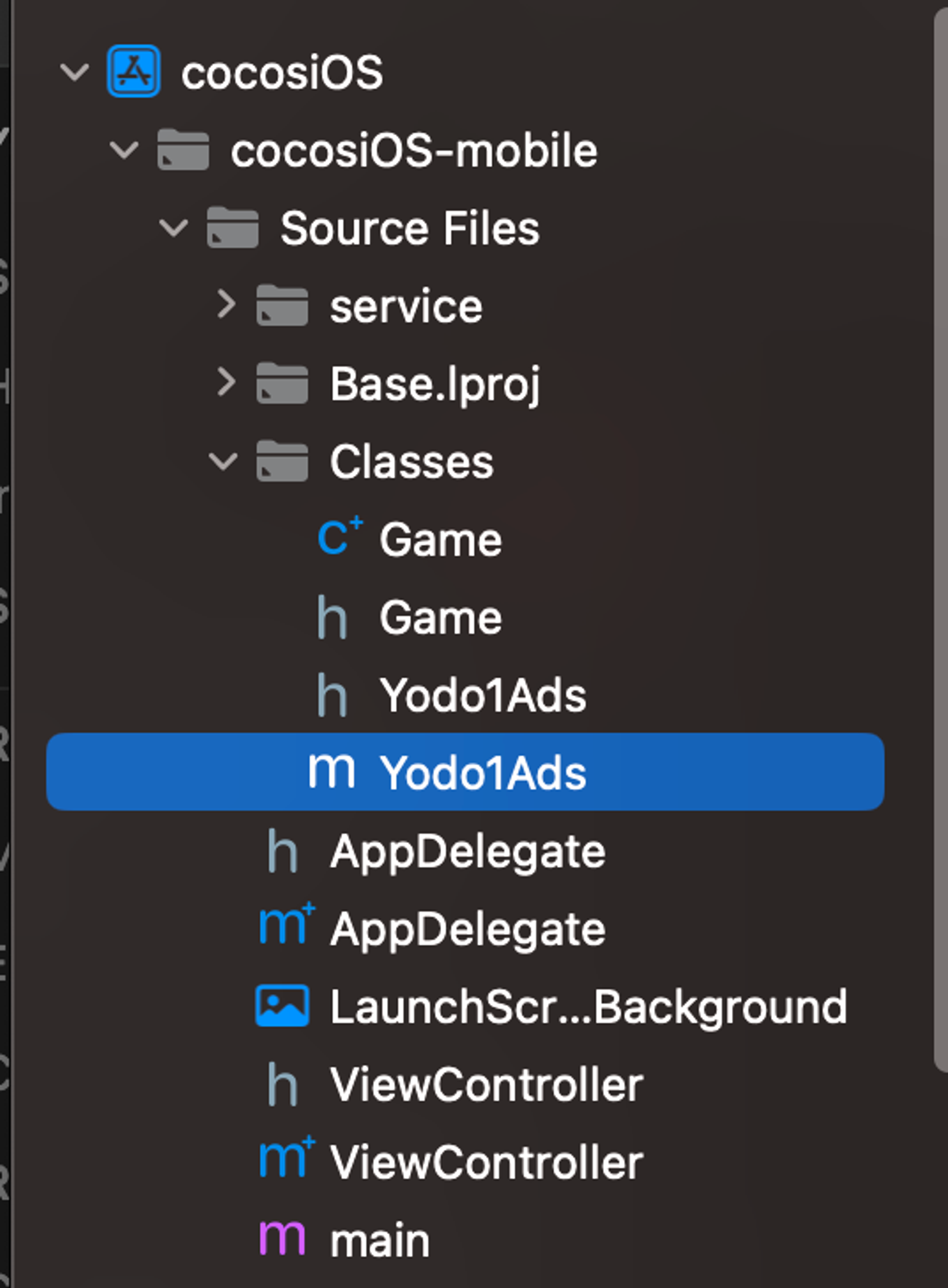
Initialize in the App delegate:
Yodo1Ads *instance = [Yodo1Ads getInstance];
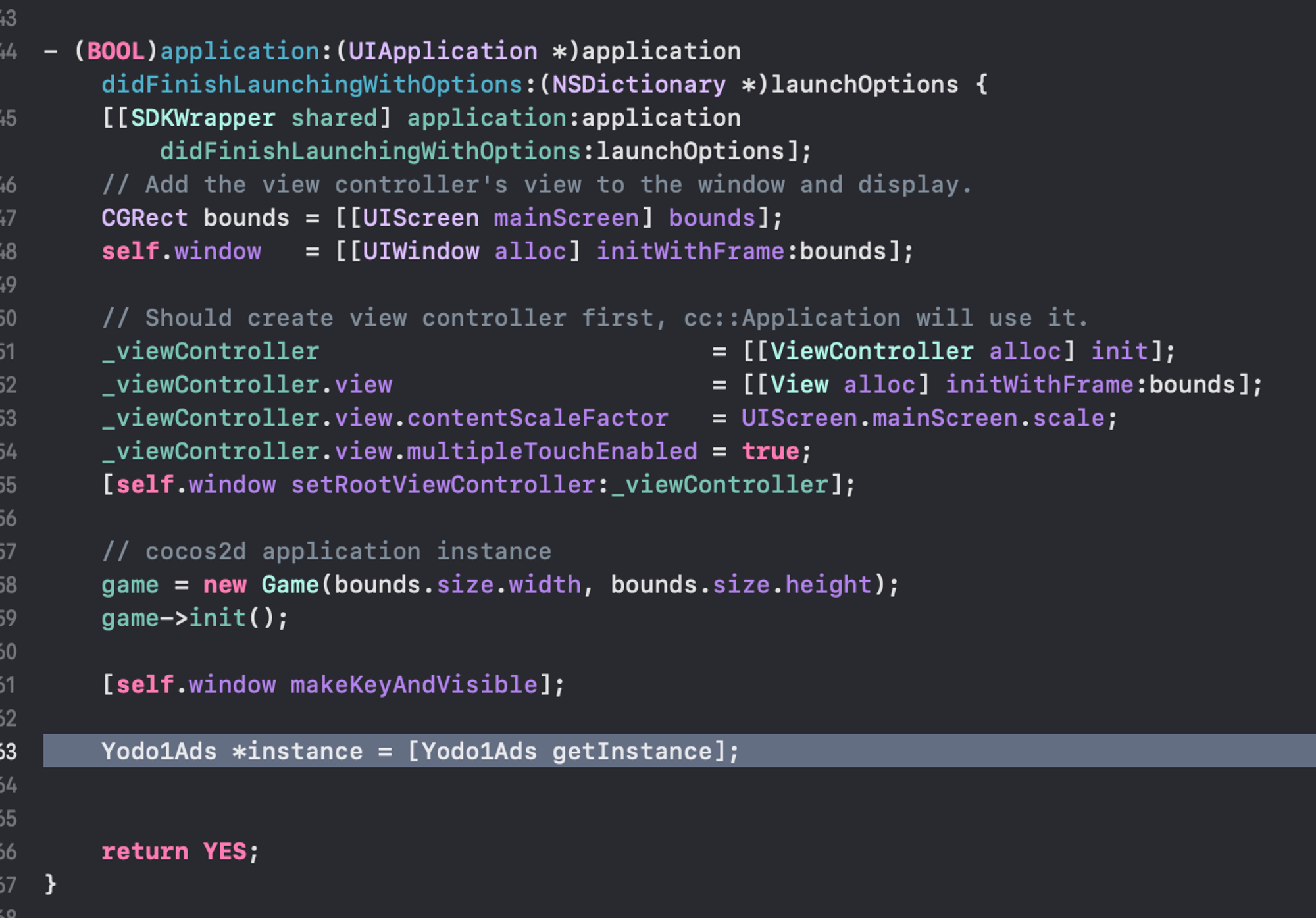
Configure the SDK
You need to add your Appkey manually in your project if you haven’t added your game’s store link in the MAS dashboard yet. You can find your app key in MAS dashboard.
Initialize MAS in your script:
import Yodo1Ads from "./Yodo1Ads";
// Initialize with built-in privacy dialog (recommended)
Yodo1Ads.getInstance().initializeMasSdk("YourAppkey", true);
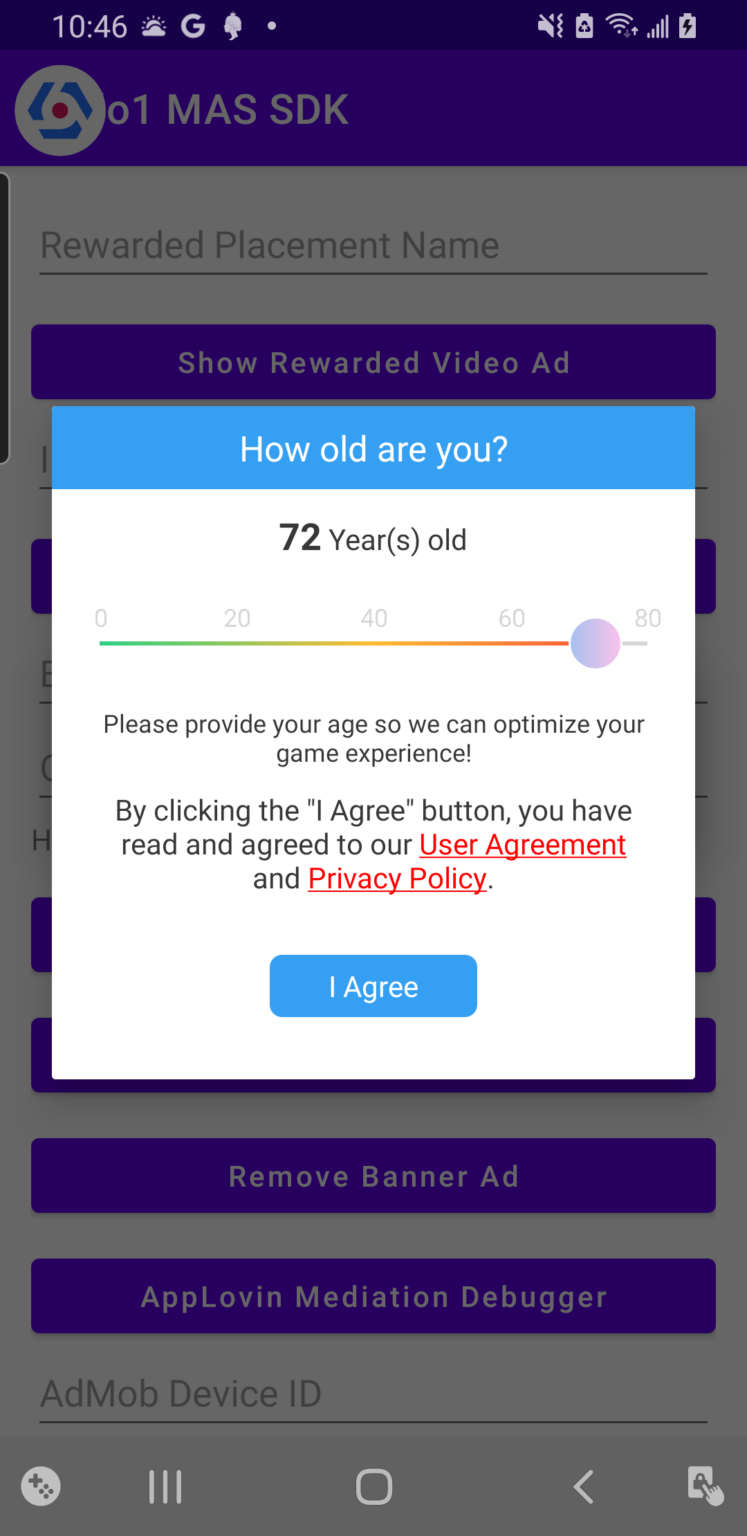
Legal Framework Configuration
We recommend using our privacy dialog for complete and automated compliance. If you choose not to use our privacy dialog, you must set the legal frameworks manually before initializing the SDK.
Configure COPPA
// For users 13 and above
Yodo1Ads.getInstance().setCOPPA(false);
// For users under 13
Yodo1Ads.getInstance().setCOPPA(true);
Configure GDPR
// For users in EEA, above 16 and who give consent
Yodo1Ads.getInstance().setGDPR(true);
// For users under 16 or who don't consent
Yodo1Ads.getInstance().setGDPR(false);
Configure CCPA
// For users in California who give consent
Yodo1Ads.getInstance().setCCPA(false);
// For users who opt out
Yodo1Ads.getInstance().setCCPA(true);
Ad Format Integration
Interstitial Ads
// Initialize
Yodo1Ads.getInstance().initializeInterstitialAds();
// Show
Yodo1Ads.getInstance().showInterstitialAds();
// Event Callbacks
public onInterstitialAdLoaded() {}
public onInterstitialAdFailedToLoad() {}
public onInterstitialAdOpened() {}
public onInterstitialAdFailedToOpen() {}
public onInterstitialAdClosed() {}
Rewarded Ads
// Initialize
Yodo1Ads.getInstance().initializeRewardAds();
// Show
Yodo1Ads.getInstance().showRewardAds();
// Event Callbacks
public onRewardAdLoaded() {}
public onRewardAdFailedToLoad() {}
public onRewardAdOpened() {}
public onRewardAdFailedToOpen() {}
public onRewardAdClosed() {}
public onRewardAdEarned() {
// Reward the user here
}
Banner Ads
// Load and show banner
Yodo1Ads.getInstance().loadBannerAds("Banner", "RIGHT", "TOP");
// Hide/Show
Yodo1Ads.getInstance().hideBannerAds();
Yodo1Ads.getInstance().showBannerAds();
// Event Callbacks
public onBannerAdLoaded() {}
public onBannerAdFailedToLoad() {}
public onBannerAdOpened() {}
public onBannerAdFailedToOpen() {}
public onBannerAdClosed() {}
Available banner sizes:
Size in dp | Description | Availability | AdSize Constant |
---|---|---|---|
320×50 | Banner | Phones and Tablets | Banner |
320×100 | Large Banner | Phones and Tablets | LargeBanner |
300×250 | IAB Medium Rectangle | Phones and Tablets | IABMediumRectangle |
Full-screen width × Adaptive height | Adaptive Banner | Phones and Tablets | AdaptiveBanner |